Asynchronous Programming — JavaScript
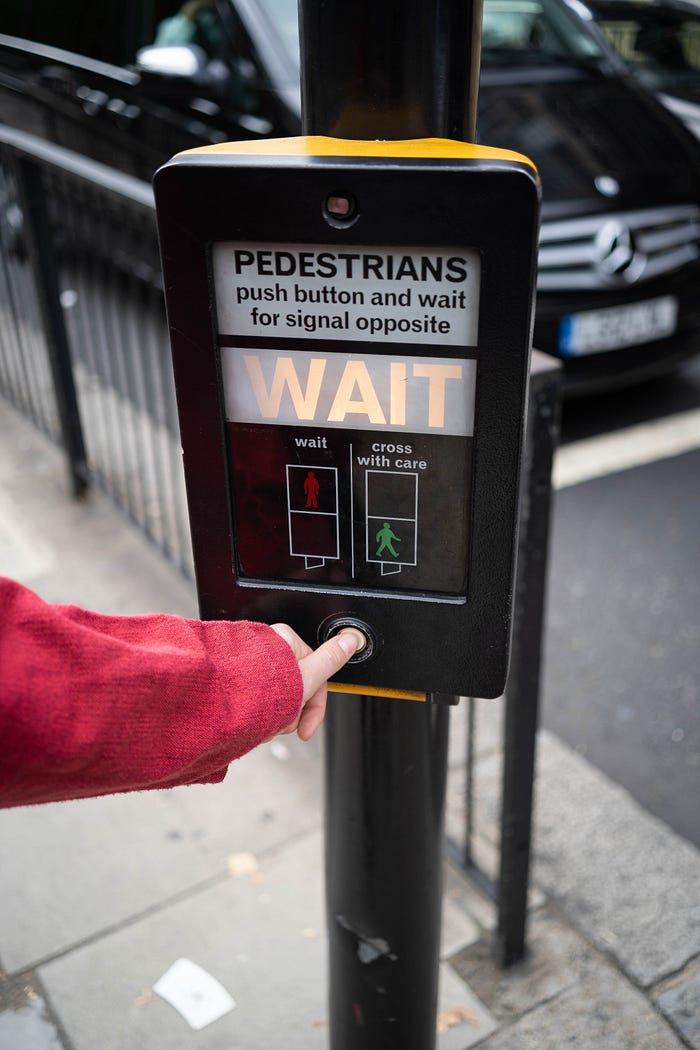
Often times in programming there will come a time when we must run a function that requires some time to complete. The problem with this is that, as the program is running this code, everything else is at a stand-still. This could pose issues for your website, application, or business, because it decreased the quality of the user experience. Luckily, there is a solution; Asynchronous Programming.
Asynchronous Programming, or async programming, is an important concept to learn for many programming languages. It is often used in modern web development to improve the performance and responsiveness of web applications. Today, We will be discussing Asynchronous Programming using JavaScript. We will discover what it is, when and why we use it, and an example of an async function using JavaScript. To start off, let’s take a look at what an Asynchronous Programming is.
What is Asynchronous Programming?
Asynchronous Programming is the process of creating async functions that are designed to perform tasks that may take some time to complete, such as waiting for a response from a server or reading a large file from a disk. When an async function is called it is returned immediately, allowing the program to continue executing code while the tasks are performed in the background.
An asynchronous function utilizes a concept called “non-blocking I/O”, which means that they do not block or wait for the task to complete before continuing to execute the next blocks of code. They instead use a callback function or a Promise object to handle the result of the task once its complete.
When is it a good idea to use Asynchronous Programming?
When discussing Asynchronous Programming, it is important to point out that there is a time and place for it. It should not be used with every function in your program, and most likely, not even half of them should be asynchronous. As explained above, they should only be used when functions are potentially long, time consuming, complicated functions that have the ability to slow down your program.
Some examples of good use cases for async programming could be querying a database located on a server, preloading data while your application starts up, or whenever you have a large number of iterations in a loop. Ultimately, if your task is simple, stick with Synchronous Programming, as sometimes it is better for your application to run step by step, but if your async functions can run seamlessly alongside the rest of your application, then go for it!
JavaScript Example
Let’s take a look at an Asynchronous function in action using JavaScript.
function resolveAfter2Seconds() {
return new Promise(resolve => {
setTimeout(() => {
resolve('resolved');
}, 2000);
});
};
async function asyncCall() {
console.log('calling');
const result = await resolveAfter2Seconds();
console.log(result);
};
asyncCall();
console.log('where will I appear?')
Here, we created a function, resolveAfter2Seconds, whose purpose is to run the callback function, resolve, after 2 seconds. We created a new instance of a Promise object to represent the eventual completion or failure of our asynchronous function. We then used setTimeout to run our callback function after 2 seconds.
Below that, we created our async function, asyncCall, to call our function. We log to the console ‘calling’ when the function is first called, we then await the response of our resolveAfter2Seconds function.
As you can see above, we then called our asyncCall function to execute our asynchronous function example. Below that, we are logging to the console. Because we are using an asynchronous function, the console.log we run after we run our asyncCall will actually run directly after ‘calling’ is printed in the console. This is because our program continued executing other code while the async function was waiting to be completed in the background. That is the power of asynchronous programming!
Conclusion
As I’m sure you can tell, our JavaScript example does not exactly need to be asynchronous, but it highlights the abilities an asynchronous function has. In this article, we discussed what async programming is, when its useful, and went over an example using JavaScript. You will likely come across Asynchronous Programming on your coding journey, and I hope this article can serve as an introduction to this concept. Keep learning, and happy coding!