Coding Challenge — Numbers Having Unique Digits
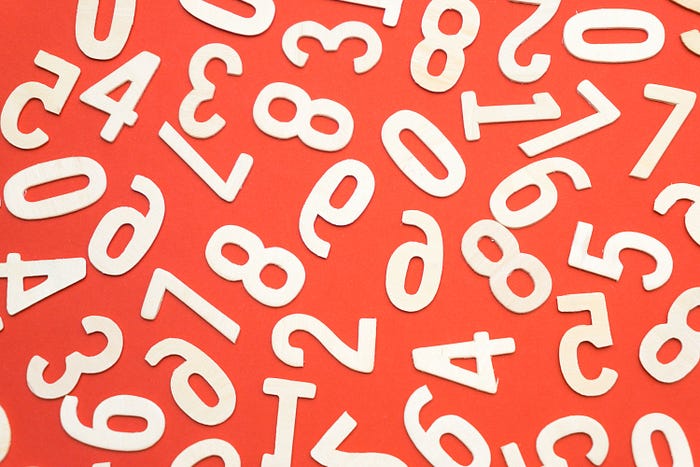
Introduction
Today, I will be discussing a coding challenge I came across the other day that stumped me for quite a bit. In coding, there will be many occasions where you find yourself saying “I don’t even know where to begin..?”, but what’s important about moments like these is that they help you become a stronger programmer. The challenge I cam across was this:
You are given an array where each element in the array is an array of two numbers. For each element in the input array, you must find the total amount of numbers between them that do not have any repeating digits, then print the total out to the console.
Example:
Input:
{{1, 10}, {1, 20}, {30, 45}}
Output:
10
19
14
Still confused? I was too… but don’t worry. We’re going to break this down.
Let’s take a look at each individual array inside the array.
First array element: {1, 10}
Numbers we are evaluating: 1 2 3 4 5 6 7 8 9 10
Without repeats: 1 2 3 4 5 6 7 8 9 10
Answer: 10
Explanation: We got the answer 10 because there are a total of 10 digits in the range of numbers, and none of them have any repeating characters.
Second array element: {1, 20}
Numbers we are evaluating: 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
Without repeats: 1 2 3 4 5 6 7 8 9 10 12 13 14 15 16 17 18 19 20
Answer: 19
Explanation: We got 19 here because there are a total of 19 digits between 1 and 20 without any repeating numbers
Third array element: {30, 45}
Numbers we are evaluating: 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
Without repeats: 30 31 32 34 35 36 37 38 39 40 41 42 43 45
Answer: 14
Explanation: Our answer is 14 because there are 14 digits ranging from 30–45 without any repeating digits
Final Answer:
10
19
14
How to Solve
Now that we’ve discussed the goal we are trying to accomplish in detail, let’s discuss how we’re going to solve it!
Step-By-Step
- Iterate through the input arr
- Establish the start and end number for our range of numbers, as well as a count of unique numbers
- create a for loop that starts with the start number, and ends after the end number
- while going through the loop, if the number is unique, increment count
Implementation
public class CountUniqueDigits {
static void printUniqueDigits(int[][] arr) {
// iterate through input array
for (int i = 0; i < arr.length; i++) {
// establish start and end of range
int start = arr[i][0];
int end = arr[i][1];
int count = 0;
// iterate through the range of numbers
for (int j = start; j <= end; j++) {
// if number is unique increment count
if (isUnique(j) == true) {
count++;
}
}
// print total count of unique numbers
System.out.println(count);
}
}
static boolean isUnique(int num) {
// initialize count as 0
int count = 0;
// compare each digit with others
// starts comparing rightmost digit with leftmost digit
while (num > 0) {
// get rightmost digit
int digit = num % 10;
// remove the rightmost digit
num = num / 10;
// assing rightmost digit to temp variable
int temp = num;
// check if rightmost digit matches leftmost digits
while (temp > 0) {
// if rightmost digit matches with any leftmost digits increase count by 1
if (temp % 10 == digit) {
count = 1;
break;
}
// remove rightmost digit from temp and get updated temp value
temp = temp / 10;
}
}
if (count == 0) {return true;}
return false;
}
public static void main(String args[]) {
int arr[][] = {{1, 10}, {1, 20}, {30, 45}};
printUniqueDigits(arr);
}
}
Conclusion
In this article, we discussed a coding challenge that deals with nested arrays, and determining if a number is unique(has no repeating digits) or not. This was quite the challenge for me. I think the hardest part was determining whether the number was unique or not. That part of the challenge really forces you to think outside of the box, which is what coding challenges are all about! I hope that by the end of this article you realize the value of coding challenges, and maybe even learned something new! Keep learning, and happy coding!